DO YOU NEED TO CALL REFPROP METHODS FROM C#, VB.NET, EXCEL VBA?
IRefProp .NET-VBA Interface is a lightweight interface to quickly and easily call REFPROP methods in C#, VB.NET, Excel VBA. It consists of an assembly file (.dll) to be used in C# and VB.NET and a type library file (.tlb) to be used in Excel VBA.
Why is the IRefProp .NET-VBA Interface necessary?
RefProp was initially written and is presently maintained in FORTRAN (since the 1970’s). It uses the Microsoft C-Runtime library to provide an interface for the FORTRAN routines to be called by native C/C++ code. However, C#, VB.NET, or Excel VBA programs can not interface directly to native code RefProp.
Sample C# Program Output
Below is sample output from a C# console application that uses IRefProp .NET-VBA Interface to compute the thermal properties of a natural gas composition.
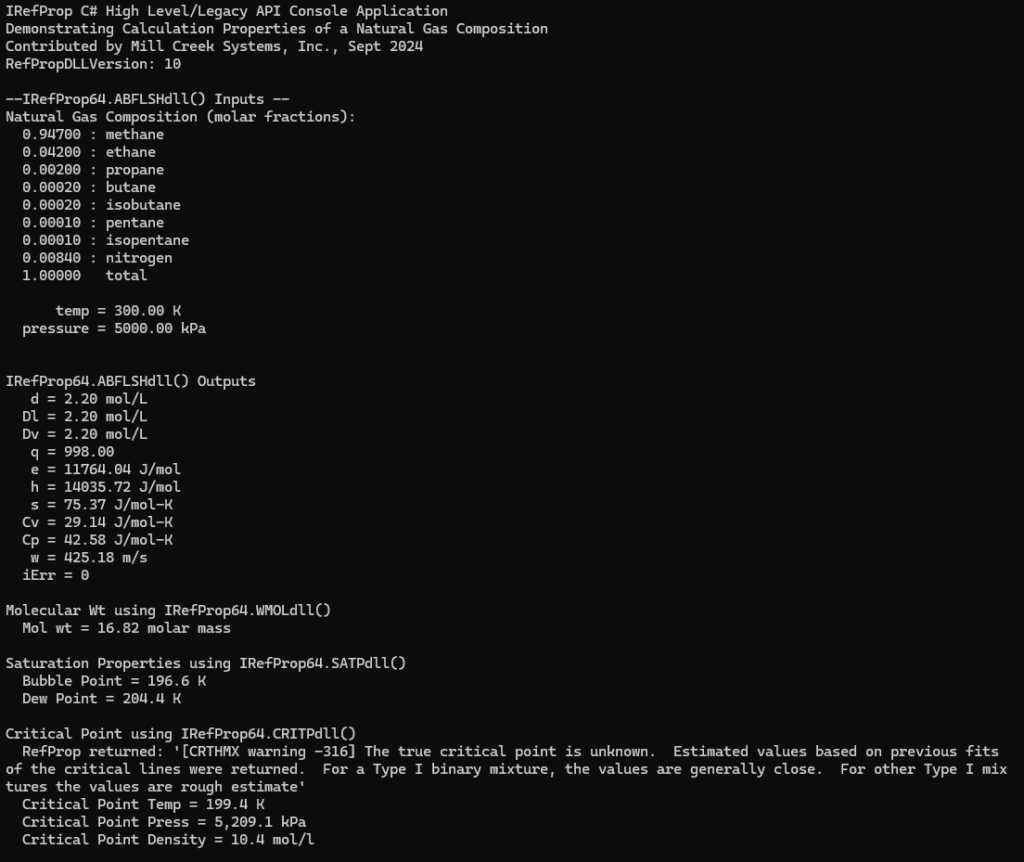
Sample Code
C#
using MCS;
Console.WriteLine("IRefProp C# High Level/Legacy API Console Application");
Console.WriteLine("Demonstrating Calculation Properties of a Natural Gas Composition");
Console.WriteLine("Contributed by Mill Creek Systems, Inc., Sept 2024");
long iErr, kph = 1;
double te = 0.0, p = 0.0, p_out = 0.0, d = 0.0, Dl = 0.0, Dv = 0.0, q = 0.0, ee = 0.0, hh = 0.0, ss = 0.0, cp = 0.0, cv = 0.0, w = 0.0;
double[] x = new double[20], xliq = new double[20], xvap = new double[20];
double[] xlkg = new double[20], xvkg = new double[20];
double tk = 0.0, tk_out = 0.0, wm = 0.0;
// get path to RefProp folder from RPPREFIX env var
string hpath = Environment.GetEnvironmentVariable("RpPrefix") + @"\fluids";
long size = hpath.Length;
// tell RefProp where the \fluids directory is. String must be 255 chars.
hpath += new String(' ', 255 - (int)size);
IRefProp64.SETPATHdll(hpath, ref size);
// create a string of fluid components to pass to RefProp
long numComps = 8;
string hfld = "methane|ethane|propane|butane|isobutan|pentane|ipentane|nitrogen";
size = hfld.Length;
hfld += new String(' ', 10000 - (int)size); // string passed to RefProp must be 10000 chars
string hfmix = "hmx.bnc" + new String(' ', 248);
string hrf = "DEF";
string herr = new String(' ', 255);
iErr = 0;
long hfldLen = hfld.Length, hfmixLen = hfmix.Length, hrfLen = hrf.Length, herrLen = herr.Length, ab_length = 2, iflag = 0;
// pass -1 to IRefProp64.SETUPdll() to get RefPRP64.dll version
numComps = -1;
IRefProp64.SETUPdll(ref numComps, ref hfld, ref hfmix, ref hrf, ref iErr, ref herr, ref hfldLen, ref hfmixLen, ref hrfLen, ref herrLen);
double version = iErr / 10000.0;
Console.WriteLine("RefPropDLLVersion: " + Convert.ToString(version));
// Ppss natural gas fluid composition component names to IRefProp64.SETUPdll()
numComps = 8;
IRefProp64.SETUPdll(ref numComps, ref hfld, ref hfmix, ref hrf, ref iErr, ref herr, ref hfldLen, ref hfmixLen, ref hrfLen, ref herrLen);
if (iErr != 0)
{
Console.WriteLine("** RefProp SETUPdll() Error = " + Convert.ToString(version));
return;
}
// Set individual molar fractions the natural gas composition. Components must add up to 1.00.
x[0] = 0.94700; // methane
x[1] = 0.04200; // ethane
x[2] = 0.00200; // propane
x[3] = 0.00020; // butane
x[4] = 0.00020; // isobutane
x[5] = 0.00010; // pentane
x[6] = 0.00010; // isopentane
x[7] = 0.00840; // nitrogen
// define temp and pressure of composition for ABFLSHdll
tk = 300; // set temp in Kelvin
p = 5000; // pressure in kPa
Console.WriteLine("");
Console.WriteLine("--IRefProp64.ABFLSHdll() Inputs --");
Console.WriteLine("Natural Gas Composition (molar fractions):");
Console.WriteLine(" " + String.Format("{0,0:0.00000}", x[0]) + " : methane");
Console.WriteLine(" " + String.Format("{0,0:0.00000}", x[1]) + " : ethane");
Console.WriteLine(" " + String.Format("{0,0:0.00000}", x[2]) + " : propane");
Console.WriteLine(" " + String.Format("{0,0:0.00000}", x[3]) + " : butane");
Console.WriteLine(" " + String.Format("{0,0:0.00000}", x[4]) + " : isobutane");
Console.WriteLine(" " + String.Format("{0,0:0.00000}", x[5]) + " : pentane");
Console.WriteLine(" " + String.Format("{0,0:0.00000}", x[6]) + " : isopentane");
Console.WriteLine(" " + String.Format("{0,0:0.00000}", x[7]) + " : nitrogen");
Console.WriteLine(" " + String.Format("{0,0:0.00000}", x[0] + x[1] + x[2] + x[3] + x[4] + x[5] + x[6] + x[7]) + " total");
Console.WriteLine("");
Console.WriteLine(" temp = " + String.Format("{0,0:0.00}", tk) + " K");
Console.WriteLine(" pressure = " + String.Format("{0,0:0.00}", p) + " kPa");
Console.WriteLine("");
string ab = "TP"; // specifies Temperature and Pressure inputs for ABFLSHdll
IRefProp64.ABFLSHdll(ref ab, ref tk, ref p, x, ref iflag, ref tk_out, ref p_out, ref d, ref Dl, ref Dv, xliq, xvap, ref q, ref ee, ref hh, ref ss, ref cp, ref cv, ref w, ref iErr, ref herr, ref ab_length, ref herrLen);
// format the results into an array of strings
string[] values = { String.Format("{0,0:0.00}", d), String.Format("{0,0:0.00}", Dl), String.Format("{0,0:0.00}", Dv), String.Format("{0,0:0.00}", q), String.Format("{0,0:0.00}", ee), String.Format("{0,0:0.00}", hh), String.Format("{0,0:0.00}", ss), String.Format("{0,0:0.00}", cp), String.Format("{0,0:0.00}", cv), String.Format("{0,0:0.00}", w), String.Format("{0,0:0}", iErr), herr };
Console.WriteLine("");
Console.WriteLine("IRefProp64.ABFLSHdll() Outputs");
Console.WriteLine(" d = " + values[0] + " mol/L");
Console.WriteLine(" Dl = " + values[1] + " mol/L");
Console.WriteLine(" Dv = " + values[2] + " mol/L");
Console.WriteLine(" q = " + values[3]);
Console.WriteLine(" e = " + values[4] + " J/mol");
Console.WriteLine(" h = " + values[5] + " J/mol");
Console.WriteLine(" s = " + values[6] + " J/mol-K");
Console.WriteLine(" Cv = " + values[7] + " J/mol-K");
Console.WriteLine(" Cp = " + values[8] + " J/mol-K");
Console.WriteLine(" w = " + values[9] + " m/s");
Console.WriteLine(" iErr = " + values[10]);
if (iErr != 0)
Console.WriteLine("hErr = " + values[11]);
Console.WriteLine("");
// calculate molecular weight of composition
Console.WriteLine("Molecular Wt using IRefProp64.WMOLdll()");
IRefProp64.WMOLdll(x, ref wm);
Console.WriteLine(" Mol wt = " + String.Format("{0:n2}", wm) + " molar mass");
// calculate saturation properties
kph = 1;
IRefProp64.SATPdll(ref p, x, ref kph, ref te, ref Dl, ref Dv, xliq, xvap, ref iErr, ref herr, ref herrLen);
Console.WriteLine("");
Console.WriteLine("Saturation Properties using IRefProp64.SATPdll()");
if (iErr == 0)
Console.WriteLine(" Bubble Point = " + String.Format("{0:n1}", te) + " K");
else
{
Console.WriteLine("IRefProp64.SATPdll() returned " + herr);
Console.WriteLine(" Bubble Point: " + "-- ");
}
// calculate saturation properties
kph = 2;
IRefProp64.SATPdll(ref p, x, ref kph, ref te, ref Dl, ref Dv, xliq, xvap, ref iErr, ref herr, ref herrLen);
if (iErr == 0)
Console.WriteLine(" Dew Point = " + String.Format("{0:n1}", te) + " K");
else
{
Console.WriteLine("IRefProp64.SATPdll() returned " + herr);
Console.WriteLine(" Dew Point: --");
}
Console.WriteLine("");
Console.WriteLine("Critical Point using IRefProp64.CRITPdll()");
// calculate critial point
double critTemp = 0.0, critPressure = 0.0, critDensity = 0.0;
IRefProp64.CRITPdll(x, ref critTemp, ref critPressure, ref critDensity, ref iErr, ref herr, ref herrLen);
if (iErr == 0)
{
Console.WriteLine(" Critical Point Temp = " + String.Format("{0:n1}", critTemp) + " K");
Console.WriteLine(" Critical Point Press = " + String.Format("{0:n1}", critPressure) + " kPa");
Console.WriteLine(" Critical Point Density = " + String.Format("{0:n1}", critDensity) + " mol/l");
}
else
{
Console.WriteLine(" RefProp returned: " + "'" + herr + "'");
Console.WriteLine(" Critical Point Temp = " + String.Format("{0:n1}" + " K", critTemp));
Console.WriteLine(" Critical Point Press = " + String.Format("{0:n1}" + " kPa", critPressure));
Console.WriteLine(" Critical Point Density = " + String.Format("{0:n1}" + " mol/l", critDensity));
}
Console.WriteLine("");
Console.WriteLine("Any Key To Exit");
Console.ReadLine();
VB.NET
Imports System
Imports MCS
Module Program
Sub Main(args As String())
Console.WriteLine("IRefProp C# High Level/Legacy API Console Application")
Console.WriteLine("Demonstrating Calculation Properties of a Natural Gas Composition")
Console.WriteLine("Contributed by Mill Creek Systems, Inc., Sept 2023")
Dim iErr As Long, kph As Long = 1
Dim te As Double = 0.0, p As Double = 0.0, p_out As Double = 0.0, d As Double = 0.0, Dl As Double = 0.0, Dv As Double = 0.0, q As Double = 0.0, ee As Double = 0.0, hh As Double = 0.0, ss As Double = 0.0, cp As Double = 0.0, cv As Double = 0.0, w As Double = 0.0
Dim x As Double() = New Double(19) {}, xliq As Double() = New Double(19) {}, xvap As Double() = New Double(19) {}
Dim xlkg As Double() = New Double(19) {}, xvkg As Double() = New Double(19) {}
Dim tk As Double = 0.0, tk_out As Double = 0.0, wm As Double = 0.0
Dim hpath As String = Environment.GetEnvironmentVariable("RpPrefix") & "\fluids"
Dim size As Long = hpath.Length
hpath += New String(" "c, 255 - CInt(size))
IRefProp64.SETPATHdll(hpath, size)
Dim numComps As Long = 8
Dim hfld As String = "methane|ethane|propane|butane|isobutan|pentane|ipentane|nitrogen"
size = hfld.Length
hfld += New String(" "c, 10000 - CInt(size))
Dim hfmix As String = "hmx.bnc" & New String(" "c, 248)
Dim hrf As String = "DEF"
Dim herr As String = New String(" "c, 255)
iErr = 0
Dim hfldLen As Long = hfld.Length, hfmixLen As Long = hfmix.Length, hrfLen As Long = hrf.Length, herrLen As Long = herr.Length, ab_length As Long = 2, iflag As Long = 0
numComps = -1
IRefProp64.SETUPdll(numComps, hfld, hfmix, hrf, iErr, herr, hfldLen, hfmixLen, hrfLen, herrLen)
Dim version As Double = iErr / 10000.0
Console.WriteLine("RefPropDLLVersion: " & Convert.ToString(version))
numComps = 8
IRefProp64.SETUPdll(numComps, hfld, hfmix, hrf, iErr, herr, hfldLen, hfmixLen, hrfLen, herrLen)
If iErr <> 0 Then
Console.WriteLine("** RefProp SETUPdll() Error = " & Convert.ToString(version))
Return
End If
x(0) = 0.947
x(1) = 0.042
x(2) = 0.002
x(3) = 0.0002
x(4) = 0.0002
x(5) = 0.0001
x(6) = 0.0001
x(7) = 0.0084
tk = 300
p = 5000
Console.WriteLine("")
Console.WriteLine("--IRefProp64.ABFLSHdll() Inputs --")
Console.WriteLine("Natural Gas Composition (molar fractions):")
Console.WriteLine(" " & String.Format("{0,0:0.00000}", x(0)) & " : methane")
Console.WriteLine(" " & String.Format("{0,0:0.00000}", x(1)) & " : ethane")
Console.WriteLine(" " & String.Format("{0,0:0.00000}", x(2)) & " : propane")
Console.WriteLine(" " & String.Format("{0,0:0.00000}", x(3)) & " : butane")
Console.WriteLine(" " & String.Format("{0,0:0.00000}", x(4)) & " : isobutane")
Console.WriteLine(" " & String.Format("{0,0:0.00000}", x(5)) & " : pentane")
Console.WriteLine(" " & String.Format("{0,0:0.00000}", x(6)) & " : isopentane")
Console.WriteLine(" " & String.Format("{0,0:0.00000}", x(7)) & " : nitrogen")
Console.WriteLine(" " & String.Format("{0,0:0.00000}", x(0) + x(1) + x(2) + x(3) + x(4) + x(5) + x(6) + x(7)) & " total")
Console.WriteLine("")
Console.WriteLine(" temp = " & String.Format("{0,0:0.00}", tk) & " K")
Console.WriteLine(" pressure = " & String.Format("{0,0:0.00}", p) & " kPa")
Console.WriteLine("")
Dim ab As String = "TP"
IRefProp64.ABFLSHdll(ab, tk, p, x, iflag, tk_out, p_out, d, Dl, Dv, xliq, xvap, q, ee, hh, ss, cp, cv, w, iErr, herr, ab_length, herrLen)
Dim values As String() = {String.Format("{0,0:0.00}", d), String.Format("{0,0:0.00}", Dl), String.Format("{0,0:0.00}", Dv), String.Format("{0,0:0.00}", q), String.Format("{0,0:0.00}", ee), String.Format("{0,0:0.00}", hh), String.Format("{0,0:0.00}", ss), String.Format("{0,0:0.00}", cp), String.Format("{0,0:0.00}", cv), String.Format("{0,0:0.00}", w), String.Format("{0,0:0}", iErr), herr}
Console.WriteLine("")
Console.WriteLine("IRefProp64.ABFLSHdll() Outputs")
Console.WriteLine(" d = " & values(0) & " mol/L")
Console.WriteLine(" Dl = " & values(1) & " mol/L")
Console.WriteLine(" Dv = " & values(2) & " mol/L")
Console.WriteLine(" q = " & values(3))
Console.WriteLine(" e = " & values(4) & " J/mol")
Console.WriteLine(" h = " & values(5) & " J/mol")
Console.WriteLine(" s = " & values(6) & " J/mol-K")
Console.WriteLine(" Cv = " & values(7) & " J/mol-K")
Console.WriteLine(" Cp = " & values(8) & " J/mol-K")
Console.WriteLine(" w = " & values(9) & " m/s")
Console.WriteLine(" iErr = " & values(10))
If iErr <> 0 Then Console.WriteLine("hErr = " & values(11))
Console.WriteLine("")
Console.WriteLine("Molecular Wt using IRefProp64.WMOLdll()")
IRefProp64.WMOLdll(x, wm)
Console.WriteLine(" Mol wt = " & String.Format("{0:n2}", wm) & " molar mass")
kph = 1
IRefProp64.SATPdll(p, x, kph, te, Dl, Dv, xliq, xvap, iErr, herr, herrLen)
Console.WriteLine("")
Console.WriteLine("Saturation Properties using IRefProp64.SATPdll()")
If iErr = 0 Then
Console.WriteLine(" Bubble Point = " & String.Format("{0:n1}", te) & " K")
Else
Console.WriteLine("IRefProp64.SATPdll() returned " & herr)
Console.WriteLine(" Bubble Point: " & "-- ")
End If
kph = 2
IRefProp64.SATPdll(p, x, kph, te, Dl, Dv, xliq, xvap, iErr, herr, herrLen)
If iErr = 0 Then
Console.WriteLine(" Dew Point = " & String.Format("{0:n1}", te) & " K")
Else
Console.WriteLine("IRefProp64.SATPdll() returned " & herr)
Console.WriteLine(" Dew Point: --")
End If
Console.WriteLine("")
Console.WriteLine("Critical Point using IRefProp64.CRITPdll()")
Dim critTemp As Double = 0.0, critPressure As Double = 0.0, critDensity As Double = 0.0
IRefProp64.CRITPdll(x, critTemp, critPressure, critDensity, iErr, herr, herrLen)
If iErr = 0 Then
Console.WriteLine(" Critical Point Temp = " & String.Format("{0:n1}", critTemp) & " K")
Console.WriteLine(" Critical Point Press = " & String.Format("{0:n1}", critPressure) & " kPa")
Console.WriteLine(" Critical Point Density = " & String.Format("{0:n1}", critDensity) & " mol/l")
Else
Console.WriteLine(" RefProp returned: " & "'" & herr & "'")
Console.WriteLine(" Critical Point Temp = " & String.Format("{0:n1}" & " K", critTemp))
Console.WriteLine(" Critical Point Press = " & String.Format("{0:n1}" & " kPa", critPressure))
Console.WriteLine(" Critical Point Density = " & String.Format("{0:n1}" & " mol/l", critDensity))
End If
Console.WriteLine("")
Console.WriteLine("Any Key To Exit")
Console.ReadLine()
End Sub
End Module
Excel VBA
'/*----------------------------------------------------------------------+
'| |
'| Copyright (c) 2002-24 Mill Creek Systems, Inc. |
'| |
'| All Rights Reserved |
'+----------------------------------------------------------------------*/
Sub Calc()
Dim IRef64 As New IRefProp64.IRefProp64_COM
'***************************************************VARIABLE DECLARATION
Dim nc, iErr, kph As Long
Dim te, p, d, Dl, Dv, q, h, ee, hh, ss, cp, cv, w, z, dPdD, d2PdD2, dPdT, dDdT, dDdP, d2PT2, d2PdTD, spare3, spare4, wmliq, wmvap, eta, tcx As Double
Dim x(0 To 19) As Double
Dim xliq(0 To 19) As Double
Dim xvap(0 To 19) As Double
Dim xlkg(0 To 19) As Double
Dim xvkg(0 To 19) As Double
Dim tstart, tstop As Double
Dim el, hl, sl, cvl, cpl, wl, etal, hjt, tcxl, ev, hv, sv, cvv, cpv, wv, etav, tcxv, qkg, wliq, wvap As Double
Dim i, phase As Integer
Dim tk, Cz, tDewPt, tBubPt, qRefProp, wm, deltaH, prevDeltaH, h_start, totalDeltaH As Double
' To-do: verify length
Dim phaseText As String * 256
Dim etaString As String * 256
Dim tcxString As String * 256
Dim hfld As String * 10000
Dim hfmix As String * 248
Dim herr As String * 255
Dim hrf As String
Dim size As Long
Dim hpath As String * 255
Dim index As Integer
Dim numComps As Long
'******************************************************************************************************
'********************************************************INITIALIZATIONS
kph = 1
te = 0#
p = 0#
d = 0#
Dl = 0#
Dv = 0#
q = 0#
h = 0#
ee = 0#
hh = 0#
ss = 0#
cp = 0#
cv = 0#
w = 0#
z = 0#
dPdD = 0#
d2PdD2 = 0#
dPdT = 0#
dDdT = 0#
dDdP = 0#
d2PT2 = 0#
d2PdTD = 0#
spare3 = 0#
spare4 = 0#
wmliq = 0#
wmvap = 0#
eta = 0#
tcx = 0#
el = 0#
hl = 0#
sl = 0#
cvl = 0#
cpl = 0#
wl = 0#
etal = 0#
hjt = 0#
tcxl = 0#
ev = 0#
hv = 0#
sv = 0#
cvv = 0#
cpv = 0#
wv = 0#
etav = 0#
tcxv = 0#
qkg = 0#
wliq = 0#
wvap = 0#
tstart = 0#
tstop = 0#
tk = 0#
Cz = 0#
tDewPt = 0#
tBubPt = 0#
qRefProp = 0#
wm = 0#
deltaH = 0#
prevDeltaH = 0#
h_start = 0#
totalDeltaH = 0#
xliq(0) = 0#
xliq(1) = 0#
xliq(2) = 0#
xliq(3) = 0#
xliq(4) = 0#
xliq(5) = 0#
xliq(6) = 0#
xliq(7) = 0#
xvap(0) = 0#
xvap(1) = 0#
xvap(2) = 0#
xvap(3) = 0#
xvap(4) = 0#
xvap(5) = 0#
xvap(6) = 0#
xvap(7) = 0#
hpath = "C:\Program Files (x86)\REFPROP\fluids"
size = Len(hpath)
'******************************************************************************************************
' Clear Debug Console
For i = 0 To 100
Debug.Print ""
Next i
Debug.Print ("IRefProp C# High Level/Legacy API Console Application")
Debug.Print ("Demonstrating Calculation Properties of a Natural Gas Composition")
Debug.Print ("Contributed by Mill Creek Systems, Inc., Oct 2024")
'****************************************************************************************
'set remainder of characters in hpath to " "
For index = 38 To 254
Mid(hpath, index) = " "
Next index
IRef64.SETPATHdll hpath, size
numComps = 8
hfld = "methane|ethane|propane|butane|isobutan|pentane|ipentane|nitrogen"
size = Len(hfld)
'set remainder of characters in hfld to " "
For index = 69 To 9999
Mid(hfld, index) = " "
Next index
hfmix = "hmx.bnc" '+ new String(' ', 248)
'set remainder of characters in hfld to " "
For index = 8 To 247
Mid(hfmix, index) = " "
Next index
hrf = "DEF"
herr = "-"
For index = 1 To 254
Mid(herr, index) = " "
Next index
iErr = 0
Dim hfldLen, hfmixLen, hrfLen, herrLen As Long
hfldLen = Len(hfld)
hfmixLen = Len(hfmix)
hrfLen = Len(hrf)
herrLen = Len(herr)
numComps = -1
IRef64.SETUPdll numComps, hfld, hfmix, hrf, iErr, herr, hfldLen, hfmixLen, hrfLen, herrLen
Dim version As Double
version = iErr / 10000#
Debug.Print ("RefPropDLLVersion: " + CStr(version))
numComps = 8
IRef64.SETUPdll numComps, hfld, hfmix, hrf, iErr, herr, hfldLen, hfmixLen, hrfLen, herrLen
'Set individual molar fractions the natural gas composition. Components must add up to 1.00.
x(0) = 0.947 '0.947 'methane
x(1) = 0.042 '0.042 'ethane
x(2) = 0.002 '0.002 'propane
x(3) = 0.0002 '0.0002 'butane
x(4) = 0.0002 '0.0002 'isobutane
x(5) = 0.0001 '0.0001 'pentane
x(6) = 0.0001 '0.0001 'isopentane
x(7) = 0.0084 '0.0084 'nitrogen
tk = 300 'CDbl(Range("B20"))
p = 5000 'CDbl(Range("B21"))
Debug.Print ("")
Debug.Print ("--IRefProp64.ABFLSHdll() Inputs --")
Debug.Print ("Natural Gas Composition (molar fractions):")
Debug.Print (" " + Format(x(0), "0.00000") + " : methane")
Debug.Print (" " + Format(x(1), "0.00000") + " : ethane")
Debug.Print (" " + Format(x(2), "0.00000") + " : propane")
Debug.Print (" " + Format(x(3), "0.00000") + " : butane")
Debug.Print (" " + Format(x(4), "0.00000") + " : isobutane")
Debug.Print (" " + Format(x(5), "0.00000") + " : pentane")
Debug.Print (" " + Format(x(6), "0.00000") + " : isopentane")
Debug.Print (" " + Format(x(7), "0.00000") + " : nitrogen")
Debug.Print (" " + Format(x(0) + x(1) + x(2) + x(3) + x(4) + x(5) + x(6) + x(7), "0.00000") + " total")
Debug.Print ("")
Debug.Print (" temp = " + Format(tk, "0.00") + " K")
Debug.Print (" pressure = " + Format(p, "0.00") + " kPa")
Debug.Print ("")
If x(0) + x(1) + x(2) + x(3) + x(4) + x(5) + x(6) + x(7) <> 1 Then
'Range("B15").Interior.ColorIndex = 3
MsgBox "Invalid Composition. Sum <> 1" & vbCrLf & "Please modify composition in ""Input Sheet"" "
'Sheets("Example").Activate
Exit Sub
Else
'Range("B15").Interior.ColorIndex = 4
End If
If iErr <> 0 Then
Range("E18") = herr
Return
End If
'ABFLSHdll
Dim ab As String
ab = "TP"
IRef64.ABFLSHdll ab, tk, p, x, iflag, tk_out, p_out, d, Dl, Dv, xliq, xvap, q, ee, hh, ss, cp, cv, w, iErr, herr, ab_length, herrLen
Debug.Print ("")
Debug.Print ("IRefProp64.ABFLSHdll() Outputs")
Debug.Print (" d = " + Format(d, "0.00"))
Debug.Print (" Dl = " + Format(Dl, "0.00"))
Debug.Print (" Dv = " + Format(Dv, "0.00"))
Debug.Print (" q = " + Format(q, "0.00"))
Debug.Print (" ee = " + Format(ee, "0.00"))
Debug.Print (" hh = " + Format(hh, "0.00"))
Debug.Print (" ss = " + Format(ss, "0.00"))
Debug.Print (" cp = " + Format(cp, "0.00"))
Debug.Print (" cv = " + Format(cv, "0.00"))
Debug.Print (" w = " + Format(w, "0.00"))
Debug.Print (" iErr = " + Format(iErr, "0"))
If iErr <> 0 Then Debug.Print ("herr = " + herr)
IRef64.WMOLdll x, wm
Debug.Print ("")
Debug.Print ("Molecular Wt using IRefProp64.WMOLdll()")
Debug.Print (" Mol wt = " & Format(wm, "0.00") & " molar mass")
Debug.Print ("")
Debug.Print ("Saturation Properties using IRefProp64.SATPdll()")
kph = 1
IRef64.SATPdll p, x, kph, te, Dl, Dv, xliq, xvap, iErr, herr, herrLen
If iErr = 0 Then
Debug.Print (" Bubble Point = " + Format(te, "0.0") + "k")
Else
Debug.Print ("IRefProp64.SATPdll() returned " & herr)
Debug.Print (" Bubble Point: " & "-- ")
End If
kph = 2
IRef64.SATPdll p, x, kph, te, Dl, Dv, xliq, xvap, iErr, herr, herrLen
If iErr = 0 Then
Debug.Print (" Dew Point = " + Format(te, "0.0") + "k")
Else
Debug.Print ("IRefProp64.SATPdll() returned " & herr)
Debug.Print (" Dew Point: " & "-- ")
End If
Dim critTemp, critPressure, critDensity As Double
critTemp = 0
critPressure = 0
critDensity = 0
Debug.Print ("")
Debug.Print ("Critical Point using IRefProp64.CRITPdll()")
IRef64.CRITPdll x, critTemp, critPressure, critDensity, iErr, herr, herrLen
If iErr = 0 Then
Debug.Print (" Critical Point Temp = " & Format(critTemp, "0.00") & " K")
Debug.Print (" Critical Point Press = " & Format(critPressure, "0.00") & " kPa")
Debug.Print (" Critical Point Density = " & Format(critDensity, "0.00") & " mol/l")
Else
Debug.Print (" RefProp returned: " & "'" & herr & "'")
Debug.Print (" Critical Point Temp = " & Format(critTemp, "0.00") & " K")
Debug.Print (" Critical Point Press = " & Format(critPressure, "0.00") & " kPa")
Debug.Print (" Critical Point Density = " & Format(critDensity, "0.00") & " mol/l")
End If
Debug.Print ("")
Debug.Print ("")
Debug.Print ("")
End Sub
Here’s a detailed Youtube video that explains how to use IRefProp .NET-VBA: